Boosting Code Readability with Scala's For Comprehension
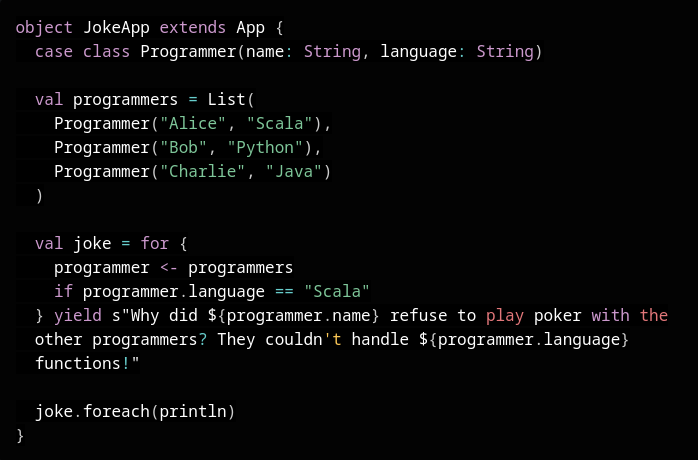
Introduction
At its core, for comprehension is a syntactic construct that allows you to write a sequence of expressions that are executed in a loop. The loop iterates over some collection, generating a value on each iteration, and applies some operations to that value. The result is a new collection that is created by combining the values generated on each iteration.
For comprehension is particularly useful when working with collections in Scala, but it can be used in a variety of other contexts as well. It provides a clean and elegant way to express complex operations on collections, making your code more readable and maintainable.
Basics of for comprehension in Scala
The syntax of for comprehension is straightforward. It consists of the for keyword, followed by a sequence of generator expressions, and an optional guard expression, followed by a yield expression. Here's an example:
val fruits = List("apple", "banana", "orange")
val result = for {
fruit <- fruits
if fruit.startsWith("a")
} yield fruit.toUpperCase
In this example, we have a List of fruits, and we use for comprehension to create a new List that contains only the fruits that start with the letter "a", and their uppercase equivalents. The generator expression is fruit <- fruits
, which means that we're iterating over the fruits List. The guard expression is if fruit.startsWith("a")
, which filters out any fruits that don't start with the letter "a". The yield expression is yield fruit.toUpperCase
, which generates a new value for each iteration by converting the fruit to uppercase.
Filter/Guard clauses
Filter/guard clauses are powerful features of for comprehension that allow you to conditionally include or exclude values from the result. Here's an example:
val numbers = List(1, 2, 3, 4, 5)
val result = for {
n <- numbers
if n % 2 == 0
} yield n * 2
In this example, we have a List of numbers, and we use for comprehension to create a new List that contains only the even numbers, multiplied by two. The generator expression is n <- numbers
, which means that we're iterating over the numbers List. The filter expression is if n % 2 == 0
, which filters out any odd numbers. The yield expression is yield n * 2
, which generates a new value for each iteration by multiplying the number by two.
Nested for comprehensions
Nested for comprehensions allow you to iterate over multiple collections and perform operations on their elements in a nested loop. Here's an example:
val numbers = List(1, 2, 3)
val letters = List("a", "b", "c")
val result = for {
n <- numbers
l <- letters
} yield s"$n$l"
In this example, we have two Lists, numbers and letters, and we use for comprehension to create a new List that contains all possible combinations of a number and a letter. The generator expressions are n <- numbers
and l <- letters
, which means that we're iterating over both Lists. The yield expression is yield s"$n$l"
, which generates a new value for each iteration by concatenating the number and letter as a string.
Using for comprehension with Option, List, and other collections
For comprehension is not limited to working with Lists. It can be used with a variety of other collections, including Option, Map, Set, and others. Here's an example using Option:
val maybeString: Option[String] = Some("hello")
val result = for { s <- maybeString } yield s.toUpperCase
In this example, we have an Option that may or may not contain a String, and we use for comprehension to create a new Option that contains the uppercase version of the String, if it exists. The generator expression is s <- maybeString
, which means that we're iterating over the Option. The yield expression is yield s.toUpperCase
, which generates a new value for each iteration by converting the String to uppercase.
Using for comprehension with Future
In Scala, Future is a type that represents a computation that may or may not have completed yet. When you have multiple futures that you want to execute in parallel, for comprehension can be a powerful tool to manage the flow of your code. Here's an example:
import scala.concurrent.Future
import scala.concurrent.ExecutionContext.Implicits.global
val futureResult: Future[Int] = for {
x <- Future { expensiveComputation1() }
y <- Future { expensiveComputation2() }
} yield x + y
In this example, we have two expensive computations that we want to run in parallel. We use for comprehension to create a new Future that will contain the sum of the results of both computations. The generator expressions are x <- Future { expensiveComputation1() }
and y <- Future { expensiveComputation2() }
, which means that we're executing both computations in parallel. The yield expression is yield x + y
, which generates a new value for each iteration by adding the results of both computations.
Why For Comprehension is Great
Scala's for comprehension is a powerful and expressive feature that makes working with collections and sequences much more elegant and concise. Unlike traditional looping constructs, for comprehension is designed to be declarative and expressive, allowing you to specify what you want to achieve instead of how you want to achieve it.
One of the key benefits of for comprehension is that it enables you to chain multiple operations together in a clear and concise way. You can use for comprehension to perform a wide range of operations on collections, such as filtering, mapping, and reducing, without having to write cumbersome and hard-to-read nested loops.
Another benefit of for comprehension is that it provides a clean and expressive syntax for working with monads, such as Option and Future (sometimes). By using for comprehension with monads, you can write code that is more readable and easier to understand, and you can avoid the need for nested if statements or try-catch blocks.
For comprehension is also great for handling complex control flow in your code. It allows you to express complex operations in a more natural and intuitive way, without sacrificing readability or maintainability.
val numbers = List(1, 2, 3, 4, 5, 6, 7, 8, 9, 10)
val result = numbers
.flatMap(x => if (x % 2 == 0) Some(x) else None)
.map(x => x * 2)
.filter(x => x > 10 && x < 20)
In this example, we have a list of numbers, and we want to perform a set of operations on them to filter out even numbers, double them, and then filter out any values greater than 20 or less than 10.
While the above is fine, it can be hard to read and understand, especially if there are even more complex operations involved. Here's how the same code can be written using for comprehension:
val numbers = List(1, 2, 3, 4, 5, 6, 7, 8, 9, 10)
val result = for {
x <- numbers
if x % 2 == 0
y = x * 2
if y > 10 && y < 20
} yield y
This code accomplishes the same goal, but in a much more concise and readable way. We use for comprehension to iterate over the list of numbers, filter out even numbers, double them, filter out values greater than 20 or less than 10, and then yield the results as a new list.
As you can see, for comprehension allows us to express complex operations in a more natural and intuitive way, without sacrificing readability or maintainability.
Conclusion
For comprehension is a powerful feature of Scala that can greatly improve the readability and maintainability of your code. With for comprehension, you can write complex operations on collections in a concise and expressive way, making your code more elegant and easy to understand. I hope this article has been helpful in introducing you to for comprehension, and that you'll find it useful in your own Scala projects.